Welcome to the Onshape forum! Ask questions and join in the discussions about everything Onshape.
First time visiting? Here are some places to start:- Looking for a certain topic? Check out the categories filter or use Search (upper right).
- Need support? Ask a question to our Community Support category.
- Please submit support tickets for bugs but you can request improvements in the Product Feedback category.
- Be respectful, on topic and if you see a problem, Flag it.
If you would like to contact our Community Manager personally, feel free to send a private message or an email.
Operator form queries
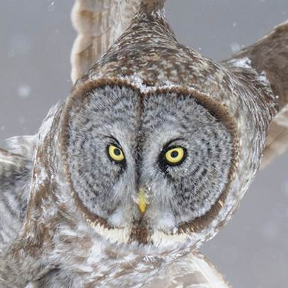
Would like to share my thoughts about compound query syntax. If you have to apply several query filters you getting coplex nested construction with arguments on the right side and subqueries on the left which is rather hard to read and edit like that:
And i decided to create an operator-form query syntax, which is based on operator overloading for (query*lambda) expression, lambda wrappers on built-in query functions, and wrapping built-in debug function in lambda that returns it's argument. Now you can use a simple, consistent syntax for compound query expressions like that:
Each wrapper returns a lambda function of built-in query with a user-defined second parameter. That labmda processes the result of evaluation of prevous functions which always is of Query type. The first equation of this conveyor should allways be of Query type as well. And finally you can add specially overloaded debug operator at any stage of evaluation, and will not brake the evaluation because internally it is wrapper over built-in debug function that calls it and then returns the argument back to evaluation process.
Some examples of code that provides these capabilities:
* operator overloading
debug function wrapping:
link to the document:
https://cad.onshape.com/documents/b2bef3c68edf40c9d2bff9cd/w/b5d571e7d6216fbb6e7b56b8/e/4e50b5b6d035682501ff1fde
qVertexAdjacent(qNthElement(qOwnedByBody(definition.testQuery,EntityType.VERTEX),1),EntityType.EDGE)and when you finnaly want to view a debug display of that query you need to assign it to separate variable.
And i decided to create an operator-form query syntax, which is based on operator overloading for (query*lambda) expression, lambda wrappers on built-in query functions, and wrapping built-in debug function in lambda that returns it's argument. Now you can use a simple, consistent syntax for compound query expressions like that:
The evaluation order was inherited from * operator and goes from left to the right (from top to bottom for vertical formatting).<code> debug(context)*<br>
qVertexAdjacent(EntityType.EDGE) *
debug(context);qOwnedByBody(definition.testQuery) *<br> qEntityFilter(EntityType.VERTEX) *<br> qNthElement(1) *
Each wrapper returns a lambda function of built-in query with a user-defined second parameter. That labmda processes the result of evaluation of prevous functions which always is of Query type. The first equation of this conveyor should allways be of Query type as well. And finally you can add specially overloaded debug operator at any stage of evaluation, and will not brake the evaluation because internally it is wrapper over built-in debug function that calls it and then returns the argument back to evaluation process.
Some examples of code that provides these capabilities:
* operator overloading
<code>lambda's
by "*" operator
export operator*(query is Query, queryFunction is function)
{
return queryFunction(query);
}//Rule for query interaction with
debug function wrapping:
//debug<br>export function debug(context is Context)<br>{<br> return function(value)<br> {<br> debug(context, value);<br> return value;<br> };<br>}lambda-wrappers for some commonly used built-in query functions:
</code><code>//return lambda for qBodyType(subquery, BodyType)<br>export function qBodyType(bodyType is BodyType)<br>{<br> return function(subquery)<br> {<br> return qBodyType(subquery, BodyType);<br> };<br>}<br><br>//return lambda for qBodyType(subquery, array of BodyType's)<br>export function qBodyType(bodyTypes is array)<br>{<br> return function(subquery)<br> {<br> return qBodyType(subquery, bodyTypes);<br> };<br>}<br><br>//return lambda for qEntityFilter(subquery, EntityType)<br>export function qEntityFilter(entityType is EntityType)<br>{<br> return function(subquery)<br> {<br> return qEntityFilter(subquery, entityType);<br> };<br>}<br><br>//return lambda for qNthElement (subquery is Query, n is number)<br>export function qNthElement(n is number)<br>{<br> return function(subquery)<br> {<br> return qNthElement(subquery, n);<br> };<br>}<br><br>//return lambda for qAttributeFilter (subquery is Query, attributePattern) returns Query<br>export function qAttributeFilter(attributePattern)<br>{<br> return function(subquery)<br> {<br> return qAttributeFilter(subquery, attributePattern);<br> };<br>}<br><br>//return lambda for qOwnedByBody (body is Query, entityType is EntityType) returns Query<br>export function qOwnedByBody(entityType is EntityType)<br>{<br> return function(body)<br> {<br> return qOwnedByBody(body, entityType);<br> };<br>}<br><br>// qVertexAdjacent (query is Query, entityType is EntityType) returns Query<br>export function qVertexAdjacent(entityType is EntityType)<br>{<br> return function(query)<br> {<br> return qVertexAdjacent(query, entityType);<br> };<br>}<br><br>// qEdgeAdjacent (query is Query, entityType is EntityType) returns Query<br>export function qEdgeAdjacent(entityType is EntityType)<br>{<br> return function(query)<br> {<br> return qEdgeAdjacent(query, entityType);<br> };<br>}<br><br>// qConstructionFilter (subquery is Query, constructionFilter is ConstructionObject) returns Query<br>export function qConstructionFilter(constructionFilter is ConstructionObject)<br>{<br> return function(subquery)<br> {<br> return qConstructionFilter(subquery, constructionFilter);<br> };<br>}<br><br>// qSketchFilter (subquery is Query, sketchObjectFilter is SketchObject) returns Query<br>export function qSketchFilter(sketchObjectFilter is SketchObject)<br>{<br> return function(subquery)<br> {<br> return qSketchFilter(subquery, sketchObjectFilter);<br> };<br>}<br><br>// qContainsPoint (subquery is Query, point is Vector) returns Query<br>export function qContainsPoint(point is Vector)<br>{<br> return function(subquery)<br> {<br> return qContainsPoint(subquery, point);<br> };<br>}<br><br>
link to the document:
https://cad.onshape.com/documents/b2bef3c68edf40c9d2bff9cd/w/b5d571e7d6216fbb6e7b56b8/e/4e50b5b6d035682501ff1fde
2
Comments
Originally I resisted the urge to do this type of thing because it is a bit of an abuse of notation, but it's hard to argue with your example -- it is certainly way more readable with the overloads. I'm going to think about putting something like that in std.
Things I would probably do a little differently / in addition:
1. I would add a QueryFilter type and tag the lambdas with it -- that way, you don't accidentally multiply with some arbitrary function.
2. I would (maybe -- I'm not certain) flip the order to be consistent with function composition -- that would require an overload of multiplying two QueryFilters
3. I would probably add + for qUnion and - for qSubtraction. qIntersection would be left without an operator, but it is much more rare (currently 26 std occurrences vs. 63 qSubtraction and 267 qUnion). The algebra doesn't quite work out (i.e., a + b - c is not the same as a - c + b) but it's close.
Initially I thought about making a function
where filter sequence has a structure like {"queryFilterName1": filterCondition1,...}. but without autocomplete of filterSequence fields this is not very usefull.
I agree with your first point. About evaluation order - it is so because i didn't ever thought that I can affect on it by overloading functions interaction, though i can find some convenience in it now.
About other query functions that combine two or more arguments of query type I don't see much use of overloading them, because it is not a big deal to have some finishing short line of code.
But appending array by += operator would be handy.
for the same thing (except b would be evaluated first), to make debug work. With this, your example with no library changes would read:
Which is about as readable as the original example and doesn't suffer from the weirdness of overloaded multiplication and left-to-right vs. right-to-left questions. At the same time, the downside is that it's suggestive of an actual method defined on the class (or worse, a lambda in some languages), rather than just a function call.
Where context doesn't need the caret since it's operating on the current Query at that point; similarly, qUnion would take one parameter or an array, and add to the query, etc. I prefer this notation a bit over the arrow notation, but that's just me.
Does Onshape currently evaluate a non-null Query as true, or throw an error? If currently an error, overloading Query & Query for intersection and Query ^ Query for exclusive-or/symmetric difference along with + and - for union and subtraction would seem to make sense. I don't see overloading the non-commutative operations as a huge problem.
PhD, Mechanical Engineering, Stanford University
By the way Kevin's approach reminds me multy-argument function curring from Haskell. Looks like there going to begin "OOP vs Functional" battle
@ilya_baran
Would you be able to use * for intersection, as that is how the QueryFilter functions would work with a Query.
Also, the code formatting on this page appears to have mucked up:
IR for AS/NZS 1100