Welcome to the Onshape forum! Ask questions and join in the discussions about everything Onshape.
First time visiting? Here are some places to start:- Looking for a certain topic? Check out the categories filter or use Search (upper right).
- Need support? Ask a question to our Community Support category.
- Please submit support tickets for bugs but you can request improvements in the Product Feedback category.
- Be respectful, on topic and if you see a problem, Flag it.
If you would like to contact our Community Manager personally, feel free to send a private message or an email.
Editing logic breaking reference to variables in array parameter?

Both of these custom features have array parameters: Texture & Freeform Spline. Input variables are lost when a new array item is added. Is there a way to fix this?
@Evan_Reese, you may be interested in the solution to this if there is one.
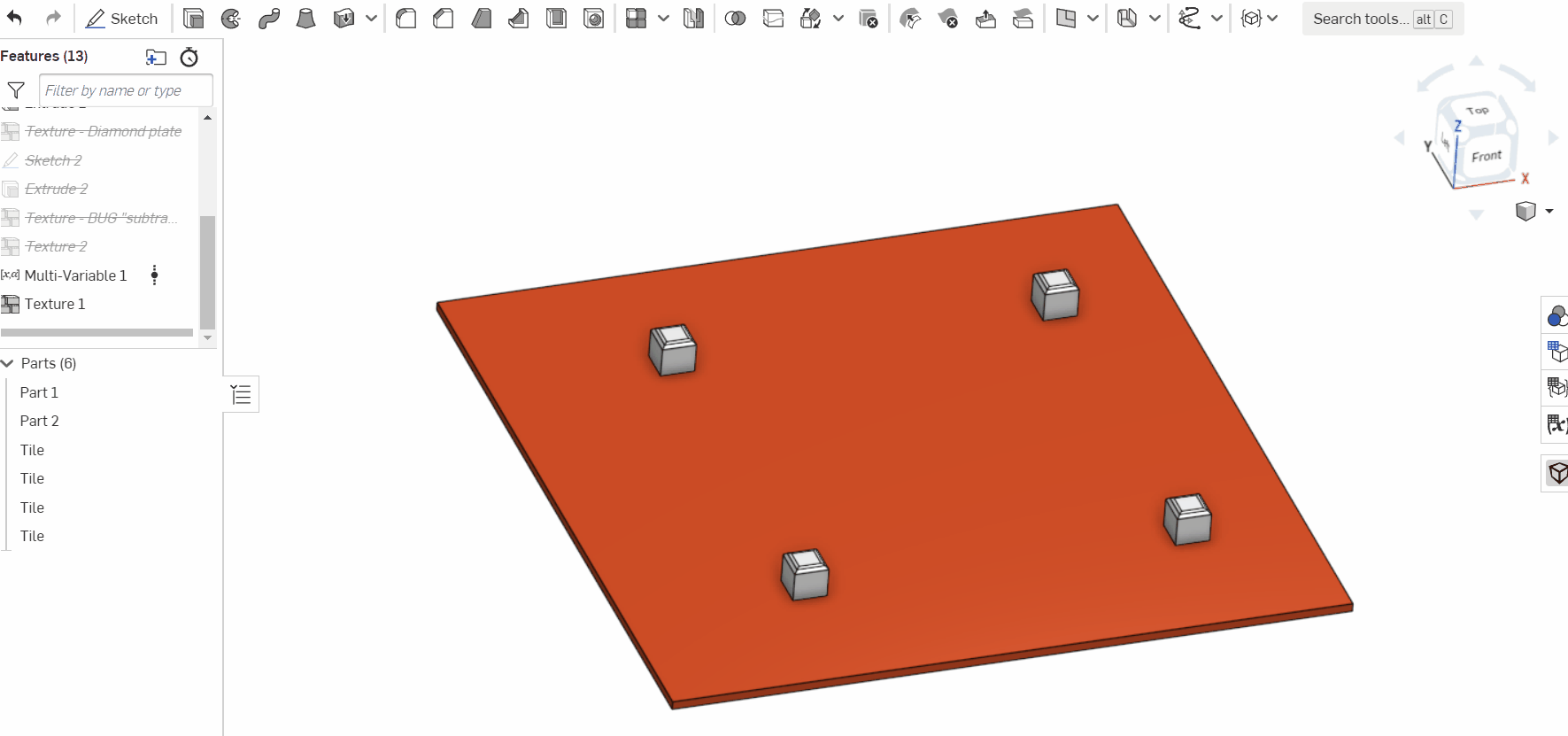
@Evan_Reese, you may be interested in the solution to this if there is one.
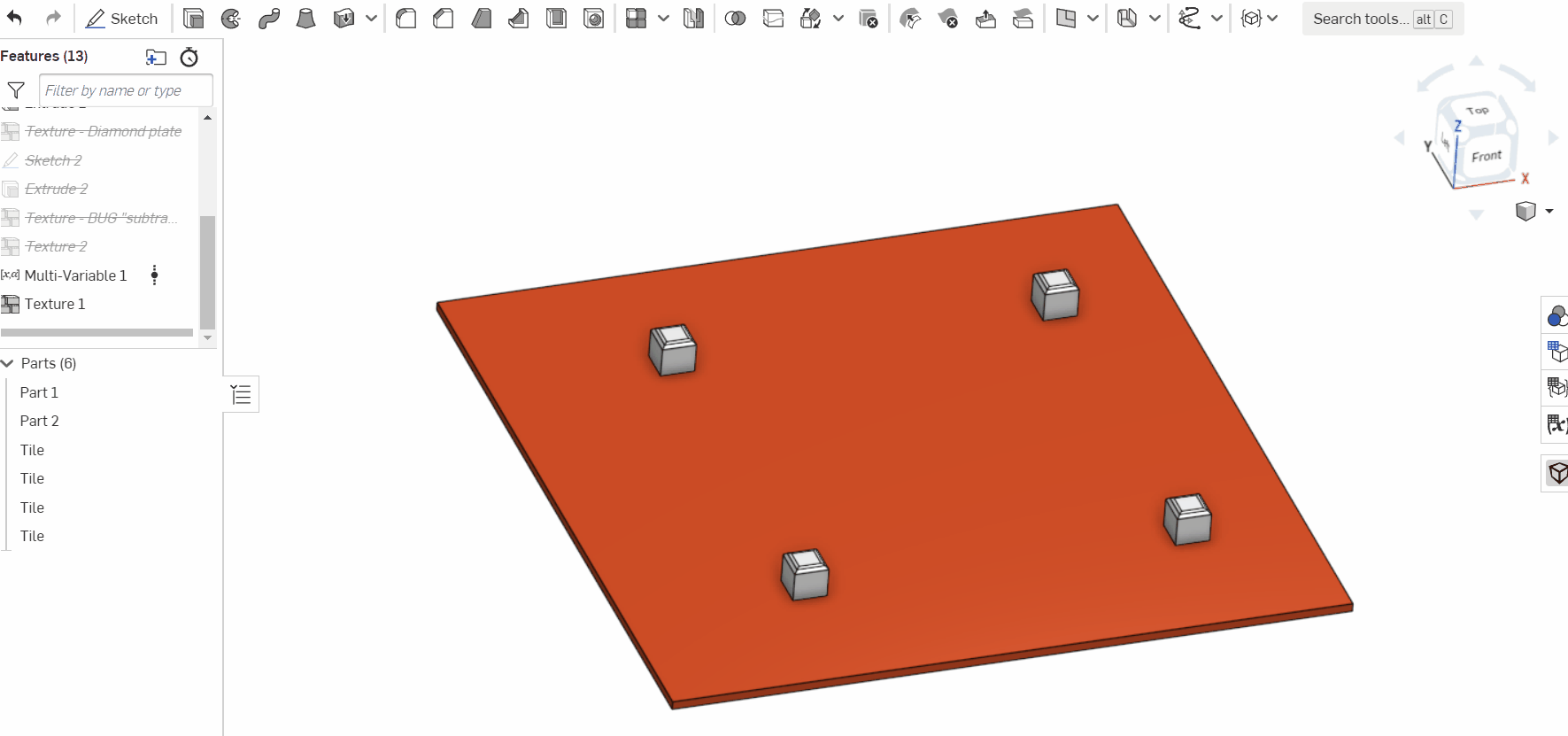
Learn more about the Gospel of Christ ( Here )
CADSharp - We make custom features and integrated Onshape apps! Learn How to FeatureScript Here 🔴
0
Best Answer
-
Alex_Kempen Member Posts: 248 EDU
The value of sortXReturned (and sortYReturned and so on) should have no bearing on whether your variables are getting overridden. Rather, it's the statement definition.groups[g].sizeX = storeSizes.x that's tripping you up, since that's setting the value of definition.groups[g].sizeX to the saved value of definition.groups[g].sizeX, which doesn't include the necessary variable information, even if the values are technically the same. The solution, therefore, is only to override the variables when the override value is actually different from the input value:// if definition.groups[g].sizeX is already the correct value, leave it alone! // if storeSizes.x is zero and autoScale.x is different from definition.groups[g].sizeX: if (storeSizes.x == 0 && !tolerantEquals(definition.groups[g].sizeX, autoScale.x)) { definition.groups[g].sizeX = autoScale.x; } else if (!tolerantEquals(definition.groups[g].sizeX, storeSizes.x)) { definition.groups[g].sizeX = storeSizes.x; }
Don't forget to repeat the following code for sizeY and sizeZ as well.CS Student at UT DallasAlex.Kempen@utdallas.eduCheck out my FeatureScripts here:2
Answers
Learn more about the Gospel of Christ ( Here )
CADSharp - We make custom features and integrated Onshape apps! Learn How to FeatureScript Here 🔴
To prevent this from happening, add conditions to shield parameters from being updated unless they actually need a new value:
I suppose this behavior could actually be used desirably to selectively break variable references or move variables to an internal parameter. Of course, that's a very specific use case, but you never know.
Near line 3201 in the Texture feature:
Learn more about the Gospel of Christ ( Here )
CADSharp - We make custom features and integrated Onshape apps! Learn How to FeatureScript Here 🔴
Also, as a more general note, using 0.999 * inch as the indicator for whether the feature has been scaled is low key not great. I would recommend using a hidden map to store that information instead - it should be more reliable and silent to the user.
I don't care too much about the name of the config so I can simply delete code at line 3158. However, I do not want to get rid of the functionality from lines 3195 and 3206. I have tried placing them in an if statement so that they only return if something has changed, however if they return at all, they break the previous groups reference to the users variables.
Line 3158, name is changed in edit logic then returned to the hidden parameter above.
Line 3195
Line 3206
Learn more about the Gospel of Christ ( Here )
CADSharp - We make custom features and integrated Onshape apps! Learn How to FeatureScript Here 🔴
Don't forget to repeat the following code for sizeY and sizeZ as well.
Here is the code that seems to be working, I will need to tweak it some more:
Learn more about the Gospel of Christ ( Here )
CADSharp - We make custom features and integrated Onshape apps! Learn How to FeatureScript Here 🔴