Welcome to the Onshape forum! Ask questions and join in the discussions about everything Onshape.
First time visiting? Here are some places to start:- Looking for a certain topic? Check out the categories filter or use Search (upper right).
- Need support? Ask a question to our Community Support category.
- Please submit support tickets for bugs but you can request improvements in the Product Feedback category.
- Be respectful, on topic and if you see a problem, Flag it.
If you would like to contact our Community Manager personally, feel free to send a private message or an email.
Options
Python API Calling Custom Features
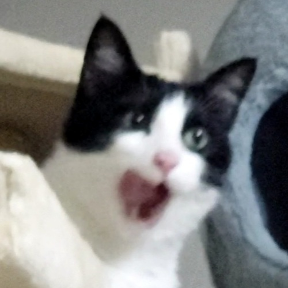
I am trying to call a custom feature using the onshape API. I can send a script to be executed successfully, but I get a 400 error if I try to add queries.
<div>import requests</div><div>import json </div><div>from APICallCreator import APICallCreator</div><div>from onshape_client.client import Client</div><div>from onshape_client.onshape_url import OnshapeElement</div><br><div>base = 'https://cad.onshape.com' # change this if you're using a document in an enterprise (i.e. "https://ptc.onshape.com")</div><div>access = '----'</div><div>secret = '----'</div><div>client = Client(configuration={"base_url": base,</div><div> "access_key": access,</div><div> "secret_key": secret})</div><br><div>class FeaturescriptCreator:</div><div> def createRequestCustom(self):</div><div> script = "function (context is Context, queries is map) {return \"hello world\";}"</div><div> queries = [{"value" : [100]}]</div><div> return script, queries</div><br><div>def callCustomFeature():</div><div> dataCreator = FeaturescriptCreator()</div><div> script, queries = dataCreator.createRequestCustom()</div><br><div> urlCreator.setRequest("featurescript")</div><div> api_url = urlCreator.getURL()</div><div> </div><div> params = {}</div><div> payload = {</div><div> "script": script,</div><div> "queries" : queries, # If I comment out this line, the call performs correctly</div><div> }</div><div> print(json.dumps(payload, indent=4))</div><br><div> headers = {'Accept': 'application/vnd.onshape.v1+json; charset=UTF-8;qs=0.1',</div><div> 'Content-Type': 'application/json'}</div><div> response = client.api_client.request(method = 'POST', </div><div> url=api_url, </div><div> query_params=params, </div><div> headers=headers, </div><div> body=payload)</div><div> parsed = json.loads(response.data)</div><div> return parsed</div><br><div>urlCreator = APICallCreator("----", "947827fa01808e74d00babf4", "273c0fd3e7a3f8c057ea3fc1")</div><div>urlCreator.setRequest("featurescript")</div><div>api_url = urlCreator.getURL()</div><br><div>headers = {'Accept': 'application/json;charset=UTF-8;qs=0.09',</div><div> 'Content-Type': 'application/json'}</div><div>params = {}</div><div>response = callCustomFeature()</div><br><div>print(json.dumps(response, indent=4, sort_keys=True))</div>This provides the following error:
onshape_client.oas.exceptions.ApiException: (400) Reason: Bad Request HTTP response headers: HTTPHeaderDict({'Date': 'Mon, 02 Jan 2023 17:55:20 GMT', 'Content-Type': 'application/json;charset=utf-8', 'Content-Length': '95', 'Connection': 'keep-alive', 'X-Request-ID': '399169b60fc62d6c564af9ed', 'Server-Timing': 'traceparent;desc="00-0000000000000000044ff6a5b4fea3f7-044ff6a5b4fea3f7-01", traceparent;desc="00-0000000000000000044ff6a5b4fea3f7-0000000000000000-01"', 'X-Api-Version': 'v1', 'On-Version': '1.157.9191.43c781405890', 'Strict-Transport-Security': 'max-age=31536000; includeSubDomains', 'X-Content-Type-Options': 'nosniff', 'X-XSS-Protection': '1; mode=block'}) HTTP response body: { "message" : "Error processing json", "moreInfoUrl" : "", "status" : 400, "code" : 0 }This error only occurs when I try to pass a value through the query field. I get the expected response if I leave the query field blank. I checked with query syntax with Glassworks and so far as I can see I entered it correctly. Not sure what I am doing wrong as the error message isn't that descriptive.
0
Comments
To work around this, you will need to edit
part_studios_api.py
In method
__eval_feature_script
use
"endpoint_path": "/api/v5/partstudios/d/{did}/{wvm}/{wvmid}/e/{eid}/featurescript",
instead of
"endpoint_path": "/api/partstudios/d/{did}/{wvm}/{wvmid}/e/{eid}/featurescript",
Note: the newer API is version 5 so
/api/v5/