Welcome to the Onshape forum! Ask questions and join in the discussions about everything Onshape.
First time visiting? Here are some places to start:- Looking for a certain topic? Check out the categories filter or use Search (upper right).
- Need support? Ask a question to our Community Support category.
- Please submit support tickets for bugs but you can request improvements in the Product Feedback category.
- Be respectful, on topic and if you see a problem, Flag it.
If you would like to contact our Community Manager personally, feel free to send a private message or an email.
Options
Geometric Variants using API, onshape-client
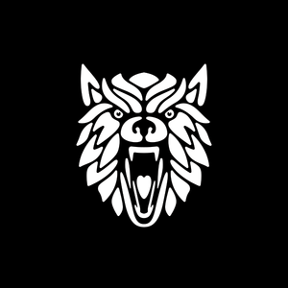
@ethan_keller924
I am finally trying to get back to this project we started about a year ago!
https://forum.onshape.com/discussion/11860/geometric-variants-derivatives-using-onshapepy-api
I was trying to get familiar with the onshape-client repo., but I am afraid I may be a little rusty.
Our code accesses a document, changes some configurations, and exports an .STL
I want to make sure we can do the same thing with onshape_clients.
Here are a few sections of the module where you could, perhaps, just point our the basic changes.
Here is the function we created to define the client
Your help is much appreciated!
I am finally trying to get back to this project we started about a year ago!
https://forum.onshape.com/discussion/11860/geometric-variants-derivatives-using-onshapepy-api
I was trying to get familiar with the onshape-client repo., but I am afraid I may be a little rusty.

Our code accesses a document, changes some configurations, and exports an .STL
I want to make sure we can do the same thing with onshape_clients.
Here are a few sections of the module where you could, perhaps, just point our the basic changes.
Here is the function we created to define the client
def connect_to_sketch( self, args ): ''' CONNECT TO ONSHAPE DOCUMENT ''' print('[{:0.6f}] Connecting to Onshape document'.format(current_time( self ))) if( len(self.did) != 24 or # Ensure inputted IDs are valid len(self.wid) != 24 or # ... len(self.eid) != 24 ): # ... raise ValueError( "Document, workspace, and element IDs must each be 24 characters in length" ) else: part_URL = "https://cad.onshape.com/documents/{}/w/{}/e/{}".format( self.did, self.wid, self.eid ) self.myPart = Part( part_URL ) # Connect to part for modification self.c = Client() # Create instance of the onshape client for exporting <br>Here is our function for exporting the STL
def export_stl( self ): ''' EXPORT STL OF GENERATED PART/VARIANT ''' print('[{:0.6f}] Export new configurations from Onshape'.format(current_time( self ))) variant_iter = self.variant_iter partname = self.partname dest = self.dst stl = self.c._api.request('get','/api/partstudios/d/{}/w/{}/e/{}/stl'.format(self.did, self.wid, self.eid)) stl_filename = ('{}{}_var{}.stl'.format(dest, partname, variant_iter)) with open( stl_filename, 'w' ) as f: # Write STL to file f.write( stl.text ) self.stl_filename = stl_filename
Your help is much appreciated!
Tagged:
1
Comments
When I execute this, I get the following error;
What is the best way of creating an OnshapeElement so that I can pass the right structure to the "part_studio_api" functions?
I was able to correct this on my program.
I also found another forum post that discussed how to use OnshapeElement() so my latest program looks like this;
Now (of course) I have different problems;
If I run the code above, I get this error
Which I believe has to do with a mismatch between the base url on the config. file (.yaml) and the url I use for the OnshapeElement function.
To solve this, I test and "fix" this I changed the address on the config file to;
The code then ran without errors, but nothing exports and writing the .STL data from the response does not generate a valid geometry file.
I am using this code for writing the response into a .STL file
So I am stuck again
I believe the issue is your base_url should be "https://cad.onshape.com" instead of "https://cad.onshape.com/documents". The end point for STL export is "https://cad.onshape.com/api/partstudios/d/{did}/{wvm}/{wvmid}/e/{eid}/stl". The way you have it written now I believe will make a call to "https://cad.onshape.com/documents/api/partstudios/d/{did}/{wvm}/{wvmid}/e/{eid}/stl" which I believe will return 404 html page.
I'm no python export, so I may be misinterpreting the code, but the STL export endpoint requires a redirect to download. What I am assuming is happening is the malformed link is giving null for a redirect URL, which the python script is following and getting an empty response.
Thanks for the response and sorry for the delay.
Correct me if I am wrong, but I think there are two ways of solving this issue. I just want to understand both so that I can 'scale-up'
First, the semi-hardcoded way, where I just have python construct the URL and send the request via the webbrowser library.
Here I do not need to use the API functions at all.
However, can anyone else run this script and download the STL?
Do other users need to have an API key?
Second, the api way, where I actually use the Onshape API to do this, is where I am having most of my issues... Here is what I have so far;
I think my problem is understanding the need and proper use of the OnshapeElement and client.part_studios_api.export_stl1() functions.
If I OnshapeElement as shown above and print the values element.did, wvm, ...etc, I get the right values.
Still, when I use the client.part_studios_api.export_stl1() function, I now get this error;
What is the proper way of using these functions?
https://github.com/onshape-public/onshape-clients/blob/0a3fa9c73e64bf6c2451a98a4c0677a0e222eca6/python/test/test_part_studios_api.py#L48
so given the error is "Access denied" I would check if your credentials match the user who has access to that document. Note that "export" permission has not been granted on that doc to anyone other than the owner as far as I can see.
Personally I found that onshape_clients was too complex to understand when debugging and unfinished (_preload_content=False.....) and just wrote my own wrapper to do the few API calls I needed using requests.
Can you elaborate on this;
Also,
Would you mind sharing an example function for how you handle requests directly?
I was trying using client.api_client.request() earlier, with 'POST' and URL as the inputs, but I get the a different error.
Here is my call;
With the error;
Thank you!
Can you sign into Onshape then go to `https://cad.onshape.com/api/users/apikeys` and validate that the API key you have set there is showing? If not, then you will have to generate/switch out the key/secret pair.
NOTE:
I know you haven't, but even while triaging issues like this, under no circumstance ever post your secret key and make sure all important data is sanitized before posting here.
This call is a `GET` not a `POST`. The error looks like a check that you are doing a `POST` with no body. If you do a `GET`, you should be getting back a redirect URL to follow to the get the STL.
<font face="Flama, sans-serif">@</font>fluvio_lobo_fenoglietto When I went to the <a href="https://cad.onshape.com/documents/4106f8fea9cf4607edeba1db/w/c11cf0ae6ab5e6297d09562d/e/3340d6f3b50b6e32e22d9a3b" title="Link: https://cad.onshape.com/documents/4106f8fea9cf4607edeba1db/w/c11cf0ae6ab5e6297d09562d/e/3340d6f3b50b6e32e22d9a3b">URL</a> and right clicked on Part1 there was no "Export" option. I just tried it again and it now has an export option so do not know what happened.<br><br>I can't share any code at current since not in a useful (standalone) state - sorry.<br><br>
My configuration file is now;
I just created a new set of keys.
When I follow the link https://cad.onshape.com/api/users/apikeys, the accessKey is displayed and the secretKey is shown as null
Either way, I get the same error... which I think refers to how I am structuring the request...?
...
I also took a side step and tried using the python requests module. I think there is something fundamental that I am not understanding about requests (in general). The following code is, again, an attempt to send the same STL request...
Here I am using onshape-client so I can extract the api_keys and pass them as payload in the GET request...
But I still get a 401 response...
Using the requests module, what is the best way of sending credentials to be able to execute the request?
Here is the working code:
The program is not completely optimized and a little redundant, but it works!
The issue I was having was resolved by the _preload_content = False input.
I was not aware that the "redirect warning" was just a "warning" instead of an error