Welcome to the Onshape forum! Ask questions and join in the discussions about everything Onshape.
First time visiting? Here are some places to start:- Looking for a certain topic? Check out the categories filter or use Search (upper right).
- Need support? Ask a question to our Community Support category.
- Please submit support tickets for bugs but you can request improvements in the Product Feedback category.
- Be respectful, on topic and if you see a problem, Flag it.
If you would like to contact our Community Manager personally, feel free to send a private message or an email.
GeometryType.OTHER_CURVE error

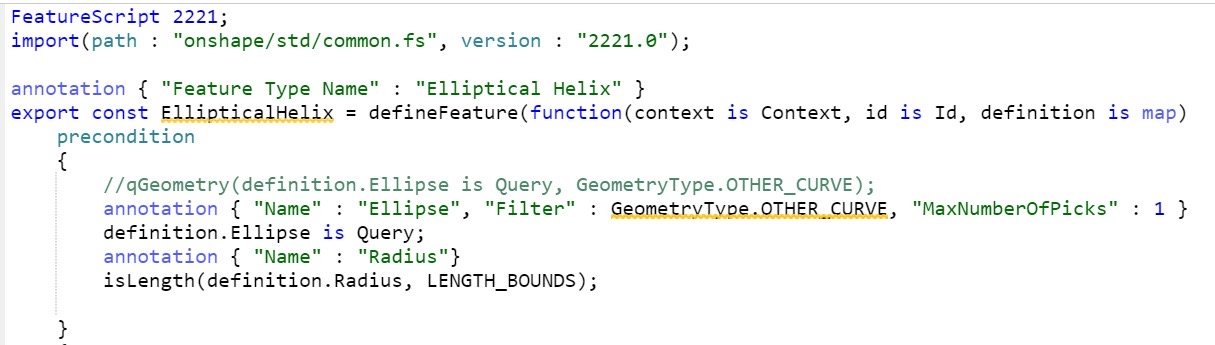
I want the input to be an ellipse. If anyone has any idea about how to get it done, you have a shoutout reserved in the script’s documentation!
0
Best Answers
-
NeilCooke Moderator, Onshape Employees Posts: 5,843
Looks like it can only be used in conjunction with qGeometry. Not sure why. You could certainly check for an ellipse using qGeometry and then throw a regenError - not very user friendly though.Senior Director, Technical Services, EMEA1 -
NeilCooke Moderator, Onshape Employees Posts: 5,843
In the body of the code, you would have to do something like:FeatureScript 2221; import(path : "onshape/std/common.fs", version : "2221.0"); annotation { "Feature Type Name" : "My Feature" } export const myFeature = defineFeature(function(context is Context, id is Id, definition is map) precondition { annotation { "Name" : "Ellipse", "Filter" : EntityType.EDGE, "MaxNumberOfPicks" : 1 } definition.ellipse is Query; annotation { "Name" : "Rad" } isLength(definition.radius, LENGTH_BOUNDS); } { const ellipse = evCurveDefinition(context, { "edge" : definition.ellipse }); if (ellipse.coordSystem == undefined || isQueryEmpty(context, qGeometry(definition.ellipse, GeometryType.OTHER_CURVE))) throw regenError("Must select an ellipse", definition.ellipse); const sketch = newSketchOnPlane(context, id + "sketch1", { "sketchPlane" : plane(ellipse.coordSystem.origin, ellipse.coordSystem.xAxis, ellipse.coordSystem.zAxis) }); skCircle(sketch, "circle", { "center" : vector(0, 0) * meter, "radius" : definition.radius }); skSolve(sketch); });
You can still get the ellipse center using evCurveDefinition.Senior Director, Technical Services, EMEA1 -
NeilCooke Moderator, Onshape Employees Posts: 5,843
It's just good practice if you don't want to accidentally overwrite a variable further down in your code. If you want to reuse a variable or update it, use var.Senior Director, Technical Services, EMEA0
Answers
You can still get the ellipse center using evCurveDefinition.